Go下time包使用及各种转换
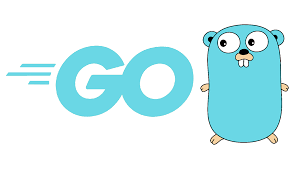
Go下time包使用及各种转换
lycheeKing时间和日期是我们编程中经常会用到的,本文主要介绍了 Go 语言内置的 time 包的基本用法。time 包提供了一些关于时间显示和测量用的函数。
1如何正确表示时间?
公认最准确的计算应该是使用“原子震荡周期”所计算的物理时钟了(Atomic Clock, 也被称为原子钟),这也被定义为标准时间(International Atomic Time)。
而我们常常看见的 UTC(Universal Time Coordinated,世界协调时间)就是利用这种 Atomic Clock 为基准所定义出来的正确时间。UTC 标准时间是以 GMT(Greenwich Mean Time,格林尼治时间)这个时区为主,所以本地时间与 UTC 时间的时差就是本地时间与 GMT 时间的时差。
1 | UTC + 时区差 = 本地时间 |
国内一般使用的是北京时间,与 UTC 的时间关系如下:
1 | UTC + 8 个小时 = 北京时间 |
在Go语言的 time 包里面有两个时区变量,如下:
- time.UTC:UTC 时间
- time.Local:本地时间
同时,Go语言还提供了 LoadLocation 方法和 FixedZone 方法来获取时区变量,如下:
1 | FixedZone(name string, offset int) *Location |
其中,name
为时区名称,offset
是与 UTC 之前的时差。
1 | LoadLocation(name string) (*Location, error) |
其中,name
为时区的名字。
1 | // 中国没有夏令时,使用一个固定的8小时的UTC时差。 |
2时间戳
时间戳是自 1970 年 1 月 1 日(08:00:00GMT)
至当前时间的总毫秒数,它也被称为 Unix 时间戳(UnixTimestamp)
1 | // timestampDemo 时间戳 |
3时间格式化
时间类型有一个自带的 time.Format
方法进行格式化,需要注意的是Go语言中格式化时间模板不是常见的Y-m-d H:M:S
,。
而是使用 2006-01-02 15:04:05.000
(记忆口诀为 6 1 2 3 4 5 )。
- 如果想格式化为12小时格式,需在格式化布局中添加
PM
。- 小数部分想保留指定位数就写0,如果想省略末尾可能的0就写 9。
1 | // formatDemo 时间格式化 |
4时间格式的转换
1 时间戳转时间字符串 (int64 —> string)
1 | timeUnix:=time.Now().Unix() //已知的时间戳 |
2 时间字符串转时间(string —> Time)
1 | formatTimeStr=”2023-02-20 13:30:39” |
3 时间字符串转时间戳 (string —> int64)
1 | // ======= 将时间字符串转换为时间戳 ======= |
4 时间戳获取日期
1 | // 获取时间,该时间带有时区等信息,获取的为当前地区所用时区的时间 |
补充
根据mysql中timestamp字段获取时间字符串
1 | 如下实例,其中lectureModel的create_time字段为timestamp |
参考资料:
[go语言时间和时间戳转换][https://www.cnblogs.com/akidongzi/p/12801574.html]
[Go语言基础之time包][https://www.liwenzhou.com/posts/Go/go-time/#autoid-1-5-0]